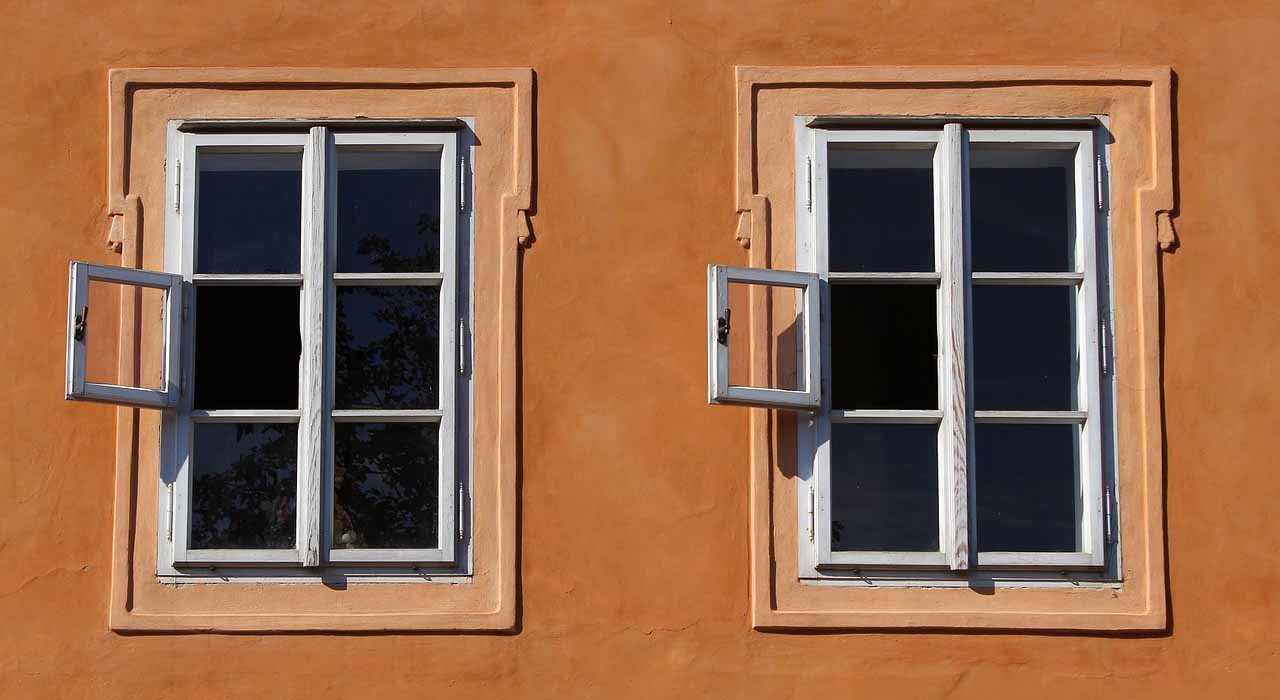
Generics in C# were introduced into the C# language with version 2 of the C# specification and the goal was to allow us to reuse more code while still being typesafe.
The type parameters in c# generics allow you to create type-safe code without knowing the final type you will be working with. In many instances, you want the types to have certain characteristics, in which case you place constraints on the type. Also, C# methods can have generic type parameters as well whether or not the class itself does.
Generics in C# allows for code reuse, as generics can parameterize the types inside of a class, interface, method or delegate. It also helps to avoid nasty problems, like typecasting and boxing.
Contents
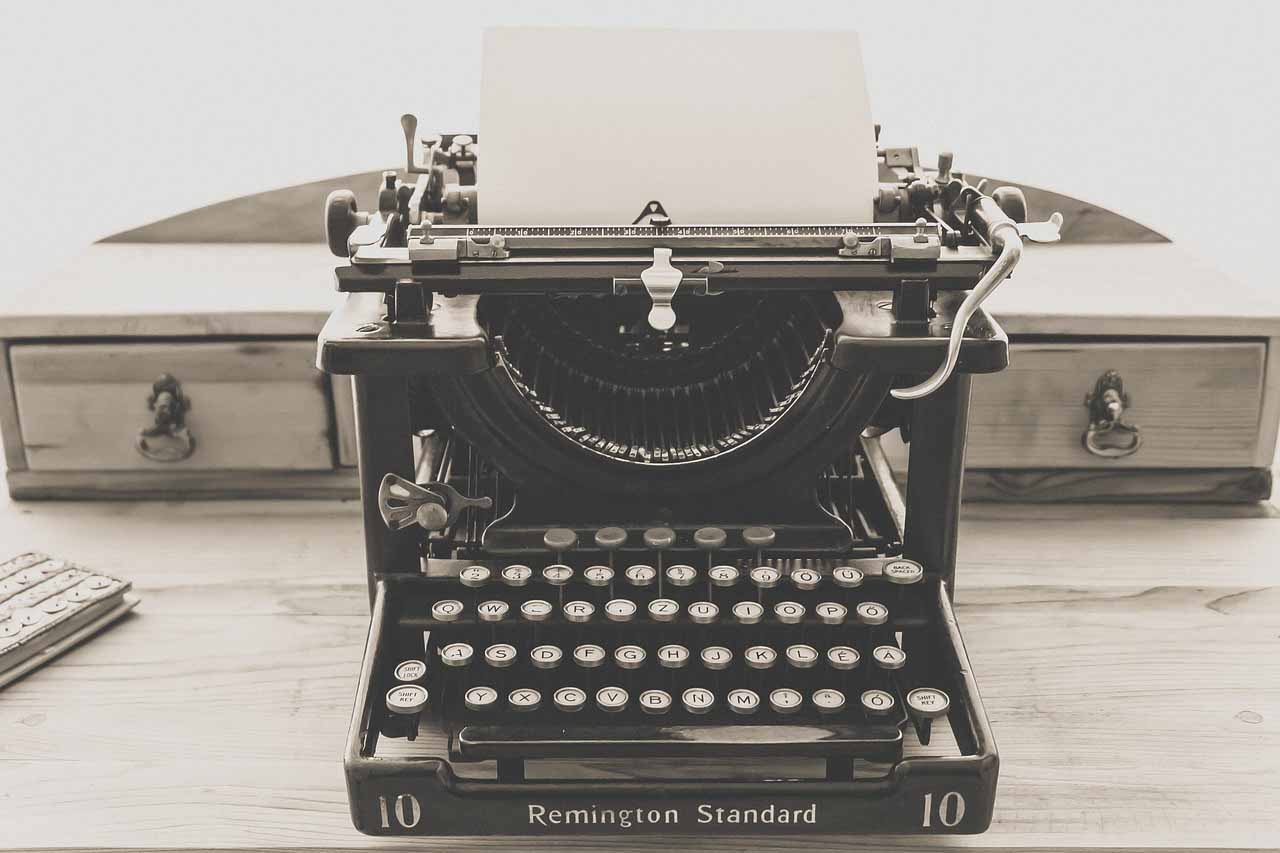
A string in C# is an object of type String whose value is text. The string object contains an array of Char objects internally. A string has a Length property that shows the number of Char objects it contains.
String is a reference type that looks like value type (for example, the equality operators == and != are overloaded to compare on value, not on reference).
In C#, you can refer to a string both as String
and string
. You can use whichever naming convention suits you. The string keyword is just an alias for the .NET Framework’s String.
Contents
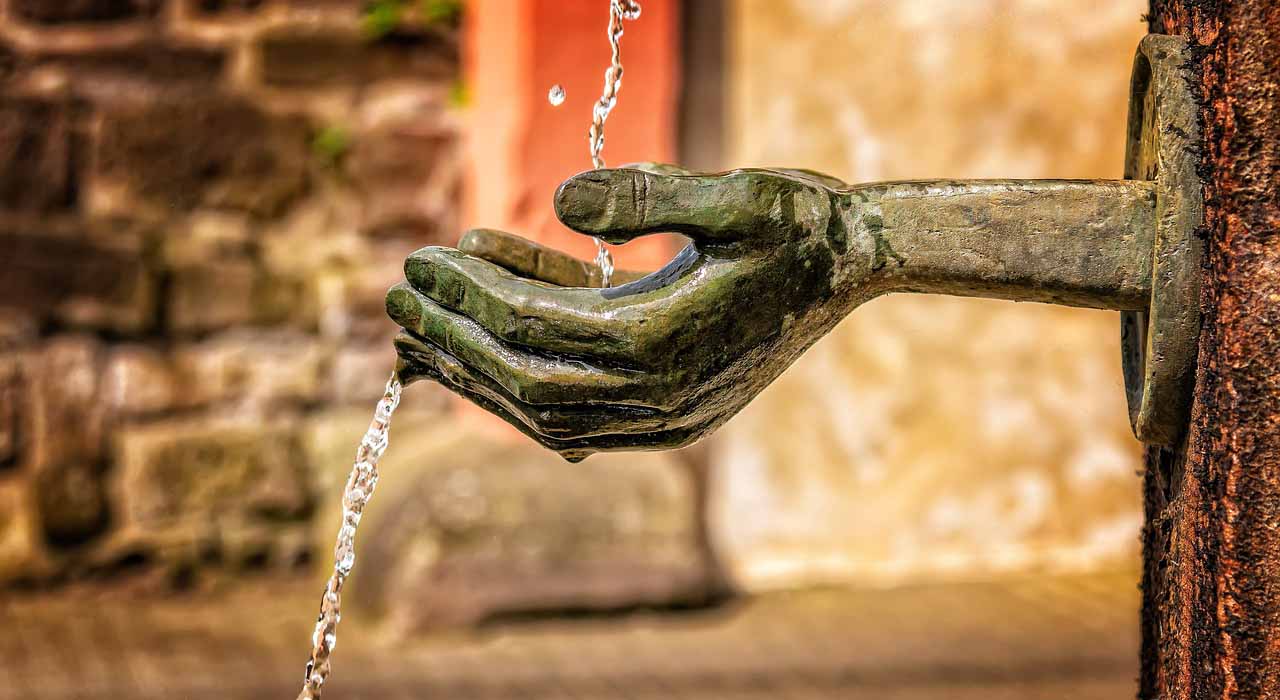
Contents
What Is A Memory Leak?
In general, a memory leak is a process in which a program or application persistently retains a computer’s primary memory. It occurs when the resident memory program does not return or release allocated memory space, even after execution, resulting in slower or unresponsive system behavior.
In unmanaged code, a memory leak is a failure to release unreachable memory, which can no longer be allocated again by any process during execution of the allocating process. This can mostly be cured by using GC (Garbage Collection) techniques or detected by automated tools.
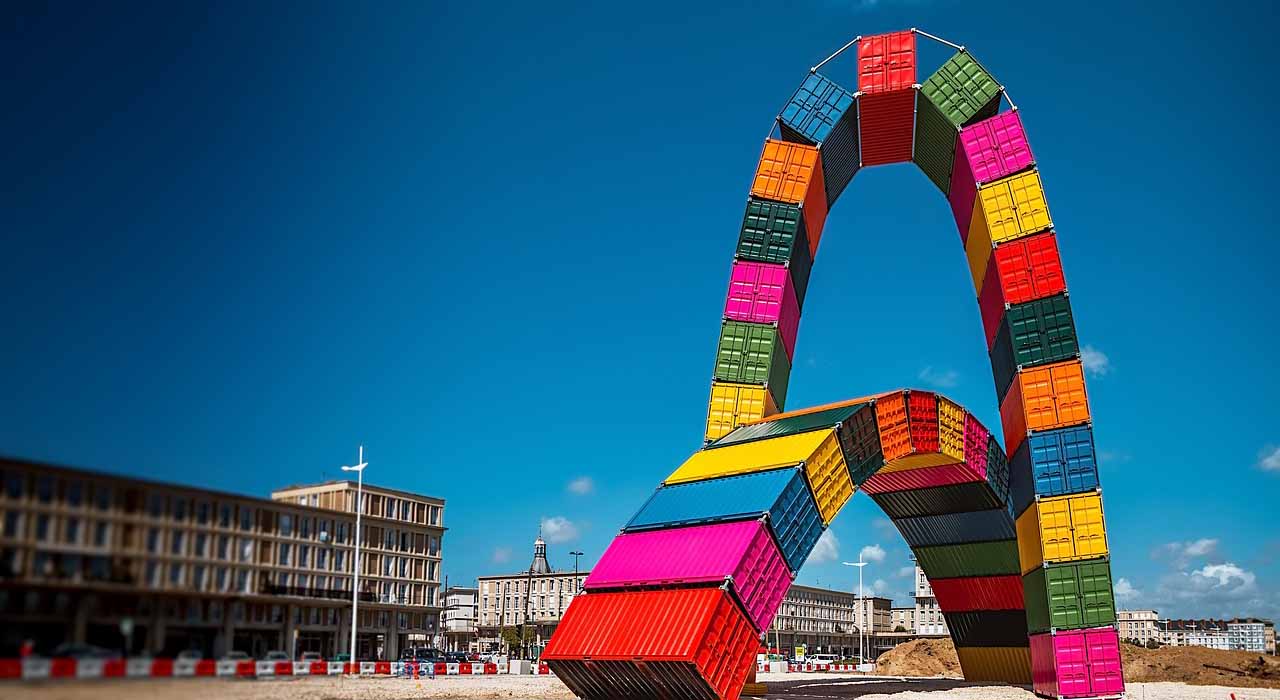
Contents
What Is Repository Pattern C# ?
A Repository mediates between the domain and data mapping layers (like Entity Framework). It allows you to pull a record or number of records out of datasets, and then have those records to work on acting like an in-memory domain object collection, and you can also update or delete records within those data set, and the mapping code encapsulated by the Repository will carry out the appropriate operations behind the scenes.