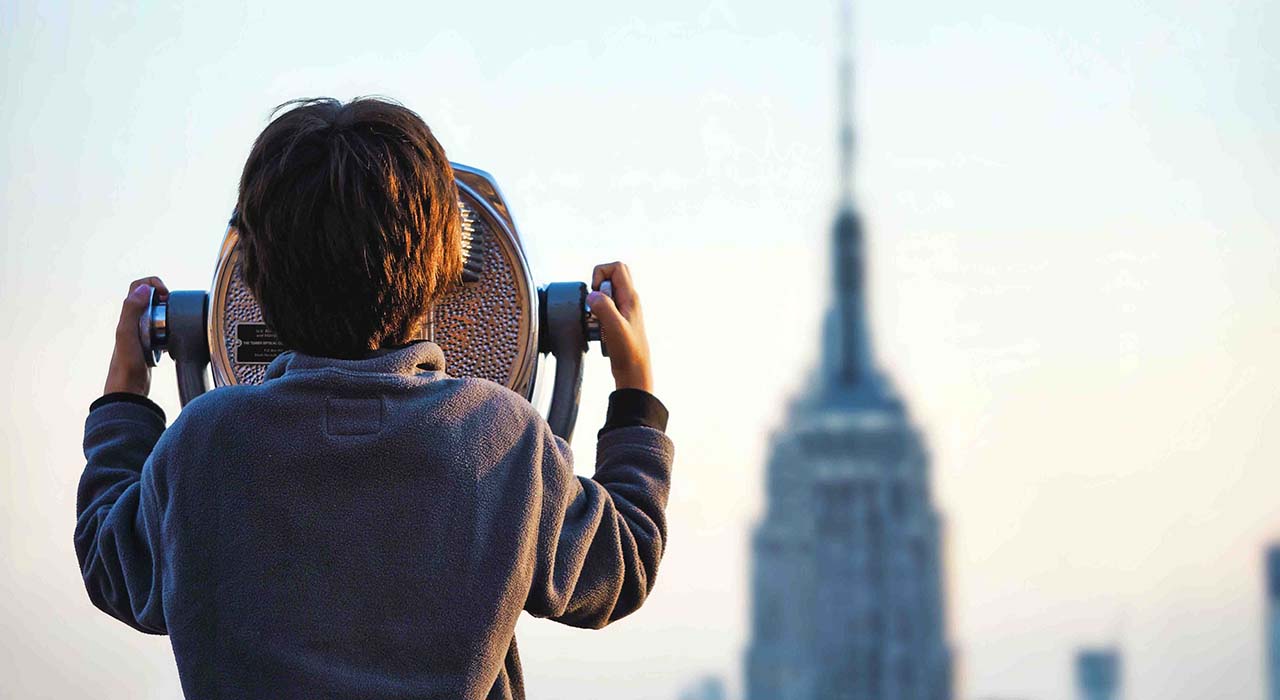
Contents
What is Observer Pattern?
Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
In this pattern, there are many observers (objects) that are observing a particular subject (also an object). Observers want to be notified when there is a change made inside
the subject. So, they register themselves for that subject. When they lose interest in the
subject, they simply unregister from the subject.
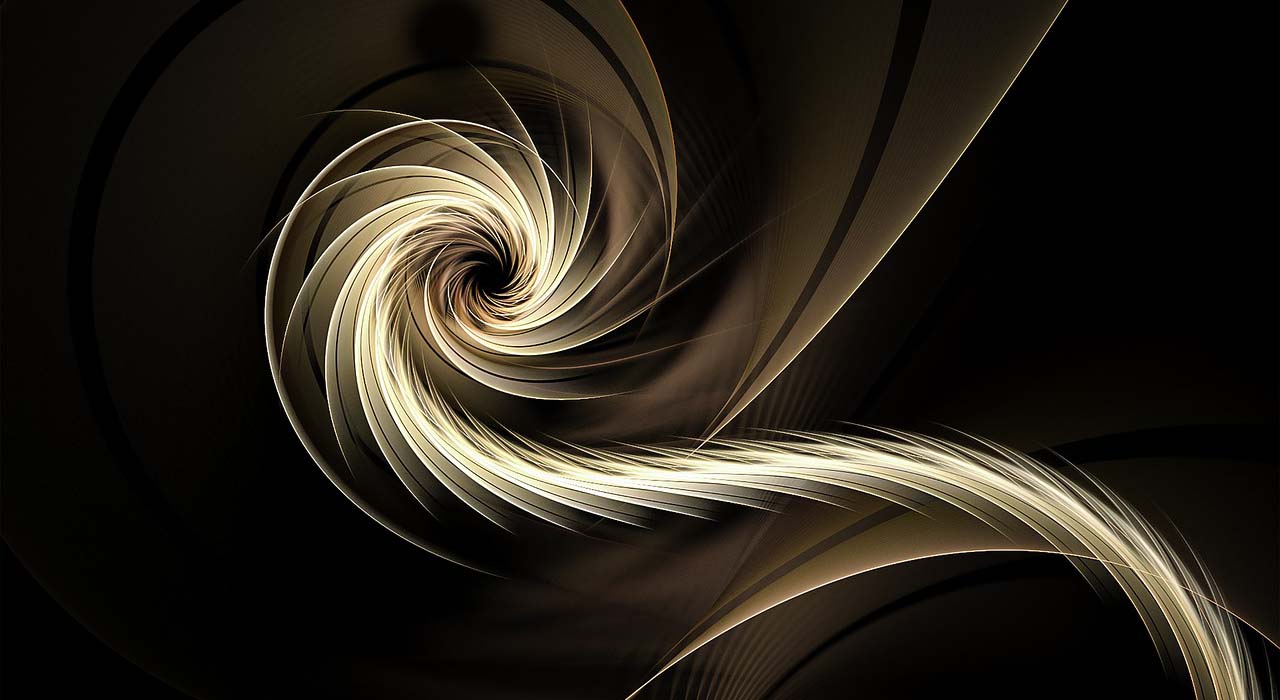
Contents
What Is Iterator Pattern?
The Iterator pattern provides a way of accessing elements of a collection sequentially, without knowing how the collection is structured.
The idea is that an aggregate object such as an array or list will give you a way to access its elements without exposing its internal structure.
Moreover, you might want to traverse the list in different ways, depending on what you want to accomplish. But you probably don’t want to bloat the List interface with operations for different traversals, even if you could anticipate the ones you will need. You might also need to have more than one traversal pending on the same list.
The Iterator pattern lets you do all this. The key idea in this pattern is to take the responsibility for access and traversal out of the list object and put it into an iterator object.
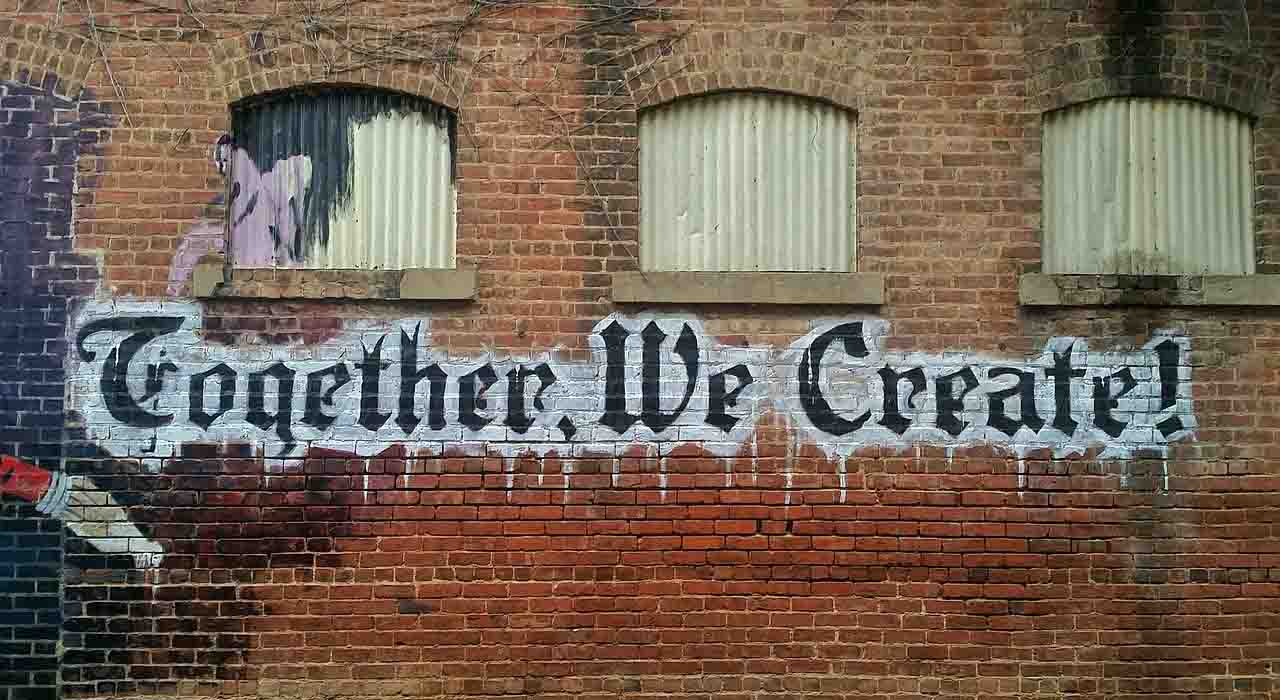
Note: If you are not familiar with String.Format and StringBuilder you can learn about it in my blog post C# String.
Recently, I saw some code that looked something like this:
StringBuilder builder = new StringBuilder();
builder.Append(String.Format("{0} {1}", firstName, lastName));
// Do more with builder...
Now, I don’t wana get into arguments about how String.Concat() is more performant here. String.Format() allows code to be more easily localized and it is being used for that purpose here. The real problem is that StringBuilder.AppendFormat() should be used instead:
StringBuilder builder = new StringBuilder();
builder.AppendFormat("{0} {1}", firstName, lastName);
// Do more with builder...